Metadata Extractors
Feature
Germain UX RUM JS extracts and collects your application’s metadata such as username or session id.
Example
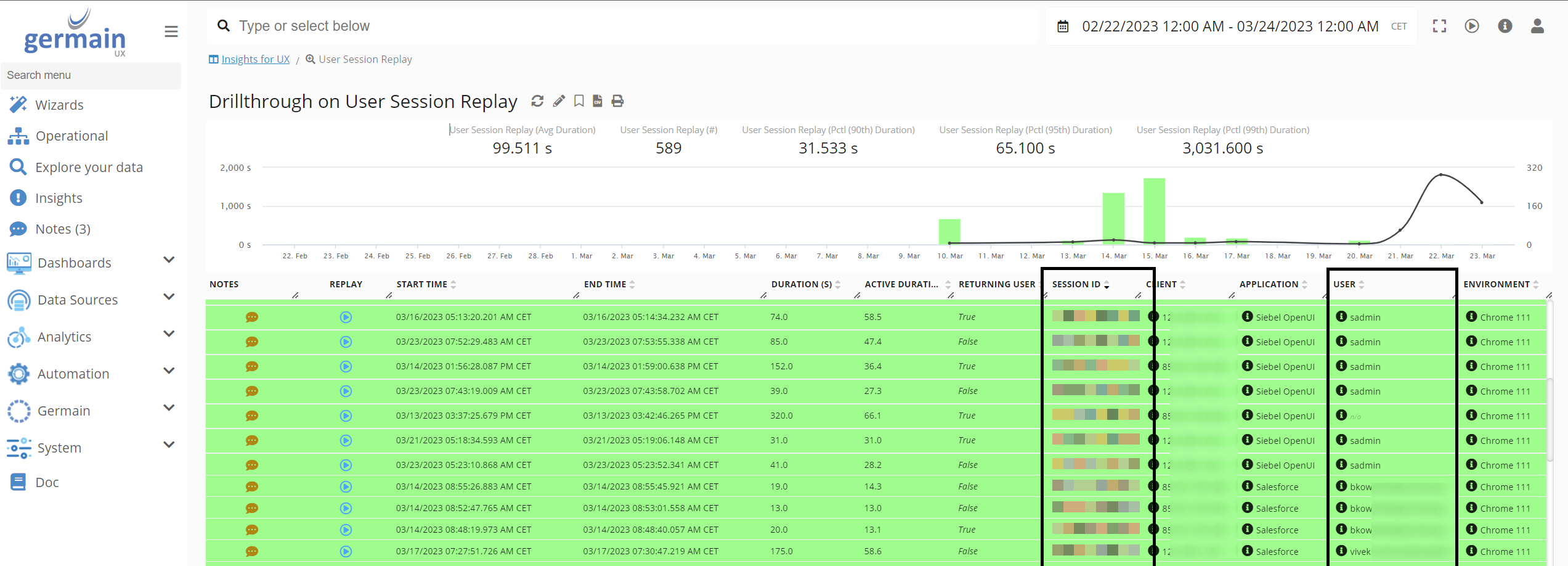
Session ID and Username Extracted Details on Drill-through
Configuration
Metadata extractor has to be configured in the Init Script. Custom function which follows the below definition has to be added to the settings.application.metadataProviders
object to extract a metadata content. It can return one of the following: value
, promise
, null
or undefined
. Undefined value won't override previously extracted value (e.g. on a different tab), but null
will.
type MetadataProviderFunction = (window: Window) => undefined | null | string | Promise<undefined | null | string>;
window
Current window.
Below example shows a custom sessionId metadata extractor where getSessionId
function contains the logic to return application’s internal session id.
const settings = germainApm.getDefaultSettings();
...
settings.application.metadataProviders['sessionId'] = getSessionId;
...
function getSessionId(window) {
...
return 'sessionIdValue';
}
...
germainApm.start(settings);
As frequently username or sessionId is not available on the page when Germain starts, we have a mechanism to wait for metadata values before we start sending UX data points which might be incomplete. Wait mechanism is configurable via settings.constants.metadataTimeoutMillis
treshold and by default it is set to 15 seconds. Please note that we will wait for all configured metadata providers to resolve and not just for the first one that returns a value. Below example shows how to extend metadataTimeoutMillis
to 20 seconds.
const settings = germainApm.getDefaultSettings();
...
settings.constants.metadataTimeoutMillis = 20 * 1000; // value must be provided in ms
...
germainApm.start(settings);
Username Extractor
One of the most common use case to define a metadata extractor is to collect your users' names available somewhere in your application.
Below example shows a username metadata extractor which looks for the logged username available on the page under a div element with username id.
const settings = germainApm.getDefaultSettings(loaderArgs, agentConfig);
...
settings.application.metadataProviders['user.name'] = (window) => {
return new Promise(resolve => { // return a Promise which will return a value
germainApm.utils.waitForElements(window, '#username', elements => { // use our utiliy function to wait for all elements available on window with a selector on the '#username'
const el = elements[0];
resolve(el.innerText || null); // return div's content meaning the username that we are looking for or null
});
});
};
...
germainApm.start(settings);
SessionId Extractor
Another frequent use case to define a metadata extractor is to collect your internal session ids.
Below example shows a sessionId metadata extractor which looks for the sessionId value inside a cookie called SESSION.
const settings = germainApm.getDefaultSettings(loaderArgs, agentConfig);
...
settings.application.metadataProviders['sessionId'] = (window) => {
return new Promise(resolve => { // return a Promise which will return a value
if(document.cookie && document.cookie.indexOf('SESSION') > -1){
const cookieItems = decodeURIComponent(document.cookie).split(';');
for (let i = 0; i < cookieItems.length; i++) {
if (cookieItems[i].trim().indexOf('SESSION') === 0) {
resolve(cookieItems[i].trim().split("=")[1]);
}
}
}
reject(null);
});
};
...
germainApm.start(settings);
Component: RUM JS
Feature Availability: 2022.1 or later